Home
About
How to ask about Qt Widgets programming problems — Example 1
Asking about fixing code that’s not compiling or behaving correctly
must be as minimal as possible, and it has to demonstrate the issue.
Why?
Because, of two main reasons:
- The help will come from other real people.
You are seeking help from other developers, volunteers, meaning they are doing it for free.
They have their own lives, joys and sorrows, messy code, and annoying colleagues to deal with.
So, minimizing your code effectively, is a way to say thank you, show respect for their time and energy, and to show appreciation for their willingness to provide the free assistance.
- It’s for your own good.
Learning how to dissect the project, extract just the problematic bits, and make a working example out of them, is an essential skill in this specialty.
The best way to acquire it, is by learning how to ask. Put yourself in the receiver’s shoes, and see if what you’re telling them is as ready as possible for them to start working on using their machines. That does not necessitate expertise; all you have to do, is to make sure other people can see the same thing you see when you run the program.
In this series, I am using random questions I find on the internet, and transform them to what they should have been. This creates a collection of practical examples to learn from.
This is Qt Widgets Minimal Example Template (unless you have to use Qt Designer):
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
return a.exec();
}
Question of the day:
How can I expand QTabWidget’s tabs?
It says:
I have three QTabWidget tabs, but there is a big margin on their right:
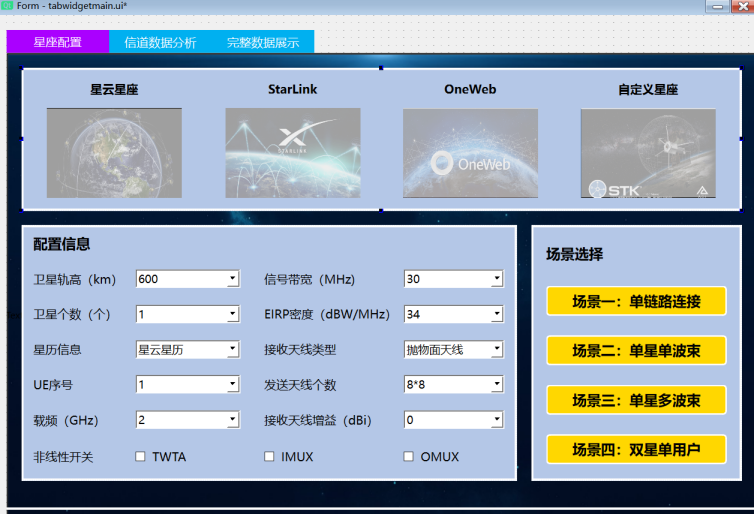
I want to remove it by expanding my three tabs.
How can I do that? Can I use the QStackedWidget to do it?
First, the screenshot; there is a lot of unrelated stuff going on there, the problem is just about the tabs.
Wouldn’t it be much cleaner, more understandable, and more importantly,
relatable, if this was used:
I got this from the official Qt Documentation,
QTabWidget
Class. It is much cleaner, and people reading the question would quickly and easily focus on the tabs.
This is still not the best option we could have, the best requires nothing but those tabs, because the content of the widgets does not matter.
So, to our Qt Widgets Minimal Example Template, we could add this:
#include <QApplication>
#include <QTabWidget>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QTabWidget tabWidget;
tabWidget.addTab(new QWidget, "tab1");
tabWidget.addTab(new QWidget, "tab2");
tabWidget.show();
return a.exec();
}
Just four more lines, one to declare the tab widget, two to add two tabs — we add two, to show that even adding more tabs does not consume that space, adding just one is not enough to demonstrate that —, and one to show the tab widget.
Notice that I did not declare the widgets used in the tab separately, as that creates more unnecessary noise. Passing a heap-allocated
QWidget
directly to
addTab
is cleaner for a minimal example, and the ownership is passed to the tab widget, so all is taken care of.
Here’s what that gets us:
A clean look, and we,
immediately, focus on the tabs.
We can even make it clearer. Annotation is a very common feature nowadays in image viewers:
How do I make the tabs take all of that space?
One clean image, one simple, short, and concise question, and we have our perfect question. You can add the code, but it serves little purpose.